Understanding the language, error messages, etc.
by Sorunome » Mon Jul 25, 2016 2:01 pm
if you draw with the color BLACK then all the pixels in your sprite will be displayed as black, if you draw with color GRAY they will be drawn gray.
So if you want a sprite with white, black and gray you erase the whole screen (make it white), draw the black parts, and then the gray parts. Or the other way round, doesn't matter.
Color INVERT is inverting the pixel, if it is white make it black and vice versa
-

Sorunome
-
- Posts: 629
- Joined: Sun Mar 01, 2015 1:58 pm
-
by Duhjoker » Mon Jul 25, 2016 9:16 pm
Wesheewww right over the head. Lol. Ok. I usually start with a blank page then do the black and then the white and then the grey. And save it. The bitmap encoder even shows the grey's but it won't print them out in grey when I copy the hex code.
I've even tried layering the sprites using a layer mapper where I can switch back and fourth from the say the grey or the black layers.
Ok I made a couple simple rooms last night just using black pixels and it looks ok. I'm using 8x8 sprites and copying them to the right places in the program I use. Turns out it's a whole lot cheaper. My first map is only 500 some of bytes.
Ok since I'm gonna use a single Sprite for each each room, I know I need to use the command gb.display.bitMap(). Do I need to use any thing in the parameters brackets. I'm pretty sure I need the name of the Sprite but I'm unsure whether I need to use the X,Y coordinates since the Sprite will take up the whole screen.
-

Duhjoker
-
- Posts: 446
- Joined: Sat Jul 02, 2016 4:57 am
- Location: Where Palm trees grow
by naed » Mon Jul 25, 2016 10:15 pm
I think I'm going to have a play around with some code tonight and try to display black/grey pixels... If I succeed I'll post my findings here (it may help)
Edit: seems easy enough to display black grey colours
here's how i did it
the black pixels i want displayed are these

- ashblack.jpg (916 Bytes) Viewed 126362 times
the code i used to display them was this
- Code: Select all
const byte ashblack[] PROGMEM = {16,16,
0xF,0xC0,
0x10,0x20,
0x20,0x10,
0x20,0x10,
0x70,0x38,
0x6F,0xD8,
0xA0,0x14,
0x84,0x84,
0x64,0x98,
0x70,0x38,
0x9F,0xE4,
0x9F,0xE4,
0x73,0x38,
0x2C,0xD0,
0x23,0x10,
0x1C,0xE0,
};
gb.display.setColor(BLACK);{
gb.display.drawBitmap(7,7,ashblack);
}
the gray pixels i want displayed are these

- ashgrey.jpg (883 Bytes) Viewed 126362 times
the code i used to display them was this
- Code: Select all
const byte ashgrey[] PROGMEM = {16,16,
0x0,0x0,
0xF,0xC0,
0x1F,0xE0,
0x1F,0xE0,
0x8,0x40,
0x0,0x0,
0x0,0x0,
0x0,0x0,
0x0,0x0,
0x3,0x0,
0x0,0x0,
0x0,0x0,
0xC,0xC0,
0x13,0x20,
0x1C,0xE0,
0x0,0x0,
};
gb.display.setColor(GRAY);{
gb.display.drawBitmap(7,7,ashgrey);
}
add both to your code and compile to see finished demo
(see below)
- Code: Select all
//imports the SPI library (needed to communicate with Gamebuino's screen)
#include <SPI.h>
//imports the Gamebuino library
#include <Gamebuino.h>
//creates a Gamebuino object named gb
Gamebuino gb;
const byte ashblack[] PROGMEM = {16,16,
0xF,0xC0,
0x10,0x20,
0x20,0x10,
0x20,0x10,
0x70,0x38,
0x6F,0xD8,
0xA0,0x14,
0x84,0x84,
0x64,0x98,
0x70,0x38,
0x9F,0xE4,
0x9F,0xE4,
0x73,0x38,
0x2C,0xD0,
0x23,0x10,
0x1C,0xE0,
};
const byte ashgrey[] PROGMEM = {16,16,
0x0,0x0,
0xF,0xC0,
0x1F,0xE0,
0x1F,0xE0,
0x8,0x40,
0x0,0x0,
0x0,0x0,
0x0,0x0,
0x0,0x0,
0x3,0x0,
0x0,0x0,
0x0,0x0,
0xC,0xC0,
0x13,0x20,
0x1C,0xE0,
0x0,0x0,
};
// the setup routine runs once when Gamebuino starts up
void setup(){
// initialize the Gamebuino object
gb.begin();
//display the main menu:
gb.titleScreen(F("My first game"));
gb.popup(F("Let's go!"), 100);
}
// the loop routine runs over and over again forever
void loop(){
//updates the gamebuino (the display, the sound, the auto backlight... everything)
//returns true when it's time to render a new frame (20 times/second)
if(gb.update()){
//prints Hello World! on the screen
gb.display.setColor(BLACK);{
gb.display.drawBitmap(7,7,ashblack);
}
gb.display.setColor(GRAY);{
gb.display.drawBitmap(7,7,ashgrey);
}
gb.display.setColor(BLACK);
//declare a variable named count of type integer :
int count;
//get the number of frames rendered and assign it to the "count" variable
count = gb.frameCount;
//prints the variable "count"
gb.display.println(count);
}
}
as you can see both sprites are being written to the same location
essentially all you have to do is write separate sprites for grey/black and overlay them on top of each other
-

naed
-
- Posts: 140
- Joined: Tue May 31, 2016 3:18 pm
by Duhjoker » Mon Jul 25, 2016 11:35 pm
Awesome!!! All right I'll check that out shortly and thank you!!!!
Ok I've run into a bug with the the Gamebuino bitmap encoder and another bitmap encoder I found. If I take my 84X48 black pixel only (background neutral) and pull it up on an encoder it gives me 9 0xFF's and a single 0xFO. At the end all the way down. It also encodes it at 88x48 for some reason.
Also still need an answer about displaying my 84x48 Sprite using go.display.bitMap. Since I'm using a single bit map Sprite do I need to put the X,Y, coordinates in the parameters and if so what numbers do I use to center it to the screen.
Now all my 8x8 sprites are coming out as 0xF0 guess i can use binary for them.
-

Duhjoker
-
- Posts: 446
- Joined: Sat Jul 02, 2016 4:57 am
- Location: Where Palm trees grow
by naed » Tue Jul 26, 2016 1:45 am
If your using a 84x48 sprite I'd use the co-ordinates 0,0 for x&y as you know this is the same size as the screen and that the x&y co-ords relate to the top left pixel
-

naed
-
- Posts: 140
- Joined: Tue May 31, 2016 3:18 pm
by Duhjoker » Tue Jul 26, 2016 2:57 am
Thats what I thought but wanted to make sure. Awesome!!!! Thank you.
Ok I think I'm starting to understand how to draw a room and display it on screen.
I'm still making my sever sprites (link), but can you "link" me to how to start adding my player sprites and how to make them move.
Or where should I start now.
-

Duhjoker
-
- Posts: 446
- Joined: Sat Jul 02, 2016 4:57 am
- Location: Where Palm trees grow
by Duhjoker » Tue Jul 26, 2016 3:32 am
Does capitalization on the hexadecimal format matter?
Really love my Gamebuino. It actually came with the acrylic cracked in a couple places so I grabbed some 1/8 inch mahogany and used the acrylic as template and made my own case. I then used a sheet of raw black walnut veneer to make it look nice. It's thinner too. Before veneering the back I cut a square hole out big enough for the battery so now the back piece carries the battery and hugs the back of the Gamebuino board.
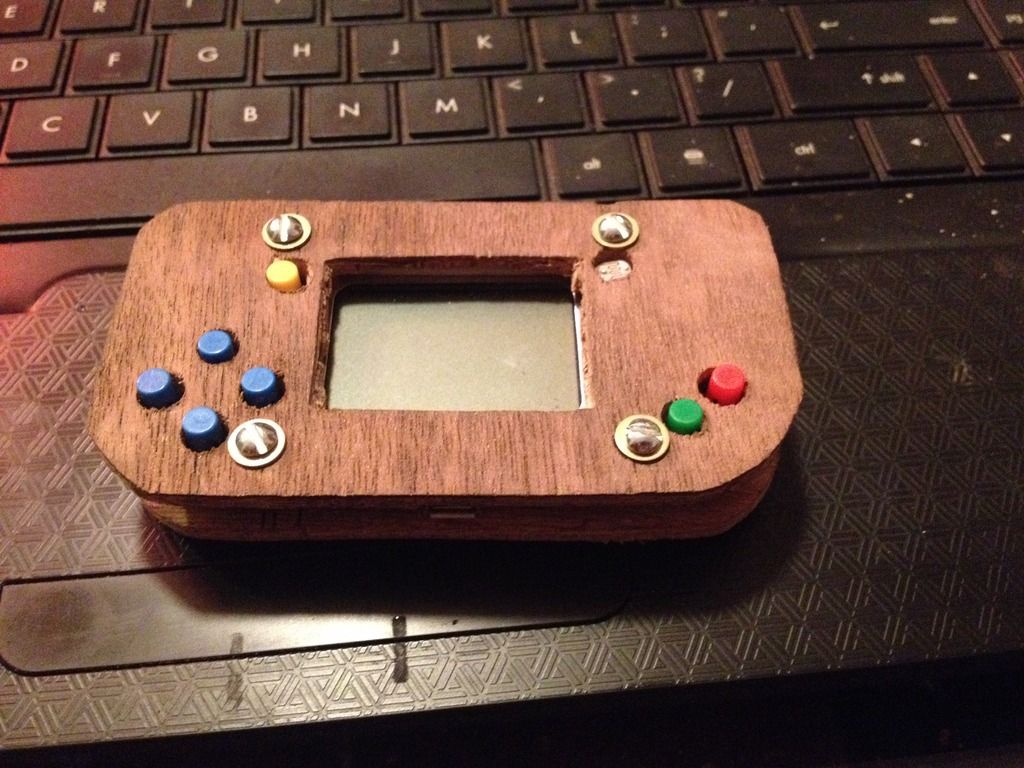
It's not perfect but I like it. Looks old fashioned.
Here's a pic showing the profile after fitting with no veneer.
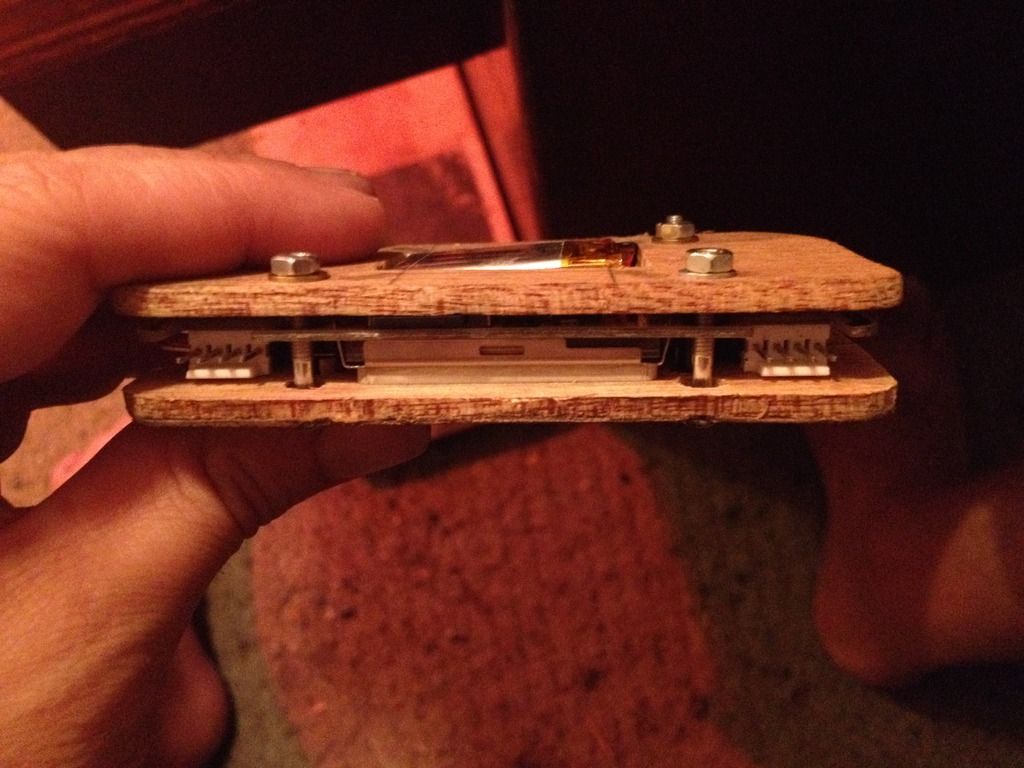
-

Duhjoker
-
- Posts: 446
- Joined: Sat Jul 02, 2016 4:57 am
- Location: Where Palm trees grow
by Duhjoker » Tue Jul 26, 2016 9:51 am
Spent the night creating 4 maps with two layers. Had to do a lot shrinking still to create maps where a 7x6 sever(link) chatacter can move through. Problem is i cant seem to come up with a 8X6 character sprite that would look good.
Is there a better example for me to use than the ball example for displaying and controlling the player sprites? Maybe one that uses more tgan one sprite, like left, right, north, south sprites for those directions.
Also on the hexadecimal does the capitolization of the last two dugits matter. Or can they be lower case?
-

Duhjoker
-
- Posts: 446
- Joined: Sat Jul 02, 2016 4:57 am
- Location: Where Palm trees grow
by Sorunome » Tue Jul 26, 2016 10:02 am
In the hex notation it doesn't matter if you use uppercase or lowercase, 0xFF is the same as 0xff (why didn't you just try this out?)
As for player direction, you make a variable which keeps track if the player is looking up/left/down/right and then display the correct sprites accordingly (This is something you should actually be able to come up with, I'm not saying everybody should be able to come up with the most optimized solution to handling this, but the general idea shouldn't be too hard to you......perhaps you should take a look at some tutorials / do some basic programming task stuff to help you out with the thought-process required for programming?)
-

Sorunome
-
- Posts: 629
- Joined: Sun Mar 01, 2015 1:58 pm
-
by naed » Tue Jul 26, 2016 8:37 pm
Lol @ Sorunome
Think you broke the record on telling someone to check out the tutorials with this thread
-

naed
-
- Posts: 140
- Joined: Tue May 31, 2016 3:18 pm
Return to Programming Questions
Who is online
Users browsing this forum: No registered users and 70 guests